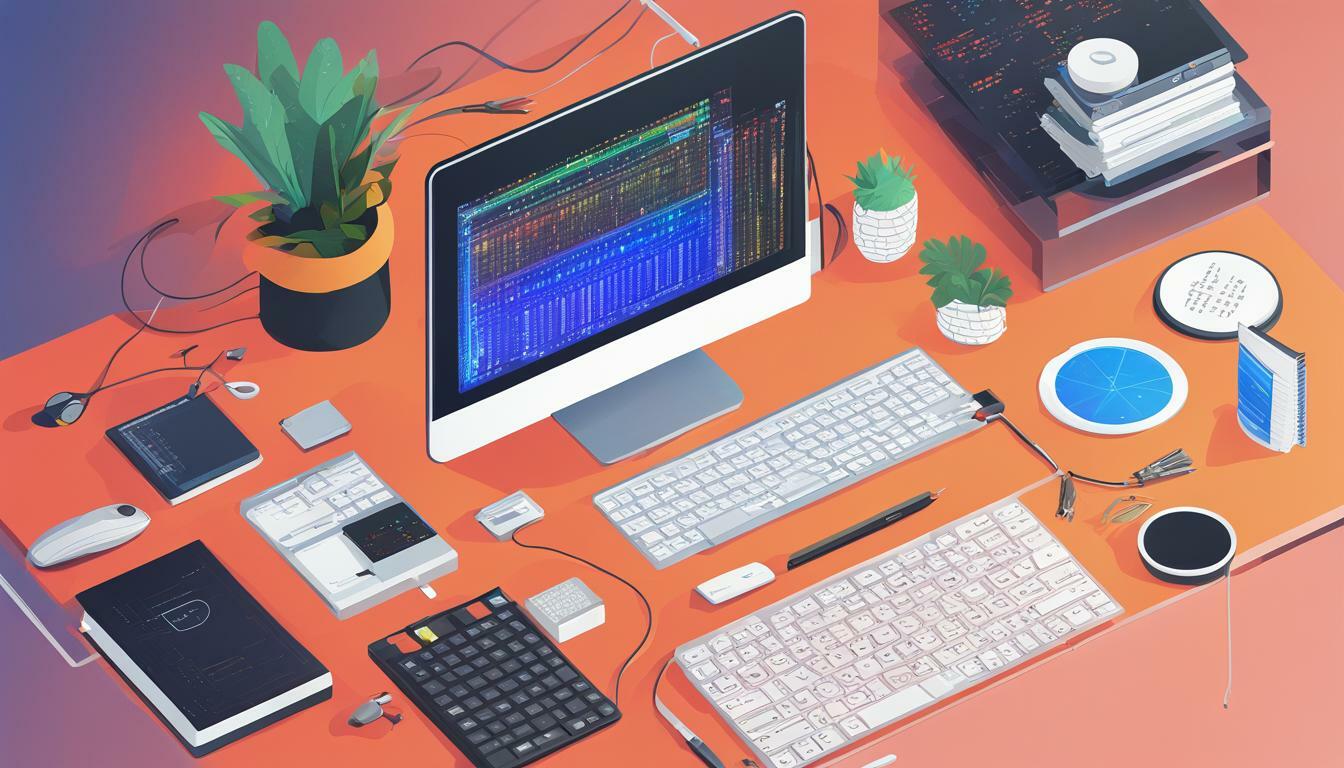
If you’re a programmer looking to enhance your coding skills and accelerate your programming journey, Typescript Code Snippets are invaluable tools that you should consider incorporating into your workflow. These code snippets provide quick and easy solutions to commonly used programming tasks and can significantly improve your coding efficiency.
In this section, we will explore the different types of Typescript Code Snippets available, such as loops, conditionals, and functions. By familiarizing yourself with these code examples and samples, you will gain a better understanding of how to leverage them in your own projects.
Exploring Typescript Code Snippets
Typescript code snippets are small portions of code that can be reused in various parts of your project. They help to simplify complex coding tasks by allowing you to write less code. Here are some Typescript code examples and samples that can be useful in your development process.
Loops
Loops are commonly used in programming to execute a particular block of code repeatedly. Here is an example of a Typescript loop:
Code | Description |
---|---|
const numbers = [1, 2, 3, 4, 5]; | This Typescript loop will print each number in the ‘numbers’ array to the console. |
Conditionals
Conditional statements are used to execute different blocks of code depending on whether a certain condition is true or false. Here is an example of a Typescript conditional statement:
Code | Description |
---|---|
function isPositive(num: number): boolean { | This Typescript function takes a number as input and returns true if the number is positive, false otherwise. |
Functions
Functions are blocks of code that can be called multiple times with different parameters. Here is an example of a Typescript function:
Code | Description |
---|---|
function addNumbers(num1: number, num2: number): number { | This Typescript function takes two numbers as input and returns their sum. |
By utilizing Typescript code samples and examples like these, you can reduce the amount of code you write and increase your coding efficiency. Stay tuned for the next section, where we will discuss how to build and organize your own Typescript code library.
Building a Typescript Code Library
As you continue to work with Typescript Code Snippets, it’s essential to start building your code library. A well-curated code library can save you time and effort by having a readily accessible repository of commonly used code snippets. It can also provide you with a place to store your personal code snippets for future use. Let’s explore how you can start building your Typescript code library.
Organizing your Code Snippets
Before building your library, it’s crucial to organize your code snippets based on their functionality. You can categorize them based on their use, such as loops, conditionals, functions, and more. This way, you can easily retrieve them when needed and avoid duplication.
Consider storing your code snippets in a folder in your local machine or cloud storage. You can also use version control tools like GitHub to store and manage your code snippets. GitHub can provide you with the added benefit of allowing you to share your code snippets with others and collaborate on projects.
Contributing to Existing Code Libraries
If you’re not up for building your code library from scratch, you can benefit from contributing to existing Typescript code libraries. Various code repositories like NPM and Github, provide a vast collection of Typescript Code Snippets. You can browse through the existing libraries and contribute your code snippets to help others and expand your coding expertise.
Discovering New Typescript Code Snippets
Aside from discovering Typescript code snippets from existing code libraries, you can also browse other resources like Stack Overflow, Medium, or Reddit to find new Typescript snippets. These resources enable you to learn from experienced developers and discover snippets that you may not have come across otherwise.
Start building your Typescript code library today and take advantage of the vast collection of existing Code Snippets. With a well-curated code library, you can save time, improve your coding efficiency, and level up your programming skills.
Advancing Your Coding Skills with Typescript Code Snippets
While Typescript code snippets can certainly assist with basic programming tasks, they can also be used to tackle more advanced coding concepts. By leveraging the power of Typescript, you can create robust applications that handle complex functionality with ease.
Error Handling with Typescript
One of the most important advanced concepts that every programmer should master is error handling. Using Typescript code snippets, you can create logic that handles different types of errors and exceptions, providing a more stable and reliable application for your end-users.
For example, the following code snippet utilizes Typescript’s built-in error handling functionality:
try {
// Code that may throw an error
} catch (error) {
// Code to handle the error
}
By using the try/catch
structure, you can test whether a block of code throws any errors during execution. If an error is detected, the catch
block is triggered, allowing you to handle the error in a way that makes sense for your application.
Object-Oriented Programming with Typescript
Another advanced concept that Typescript code snippets can help you with is object-oriented programming (OOP). By utilizing OOP principles in your code, you can create classes and objects that interact with each other in a more efficient and organized way.
For example, the following code snippet creates a basic Typescript class:
class Person {
name: string;
age: number;constructor(name: string, age: number) {
this.name = name;
this.age = age;
}sayHello() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
By creating a class like this, you can create multiple instances of the same object with unique properties. This can help you organize your code in a more logical and scalable way, allowing you to build more complex applications with less effort.
Asynchronous Programming with Typescript
A final advanced concept that you can tackle with Typescript code snippets is asynchronous programming. By using asynchronous programming techniques, you can create code that performs multiple actions simultaneously, resulting in a more responsive and efficient application.
For example, the following code snippet uses Typescript’s built-in async/await
functionality:
async function fetchUserData(userID: string) {
const response = await fetch(`/api/user/${userID}`);
const userData = await response.json();return userData;
}
By using async/await
, you can create code that waits for asynchronous actions to complete before moving on to the next step. This can help you create code that performs better and scales more efficiently, especially when dealing with large datasets or network requests.
By mastering these advanced Typescript coding concepts, you can take your programming skills to the next level and build more complex and powerful applications.
Conclusion
Typescript Code Snippets are essential to every programmer’s toolkit. By using code snippets, you can speed up your programming process and work more efficiently. Incorporating Typescript code examples and samples into your workflow can help you gain a better understanding of how to leverage the language’s features and build more complex programs.
Building a Typescript code library is crucial for maintaining your code snippets and keeping them organized. By storing your code snippets in a centralized location, you can easily access them whenever you need them and share them with your team members.
As you advance your programming skills, you can use more advanced Typescript code snippets. These snippets can help you tackle more complex programming challenges, including techniques like error handling and object-oriented programming.
Overall, Typescript Code Snippets are a valuable resource for programmers of all skill levels. By exploring Typescript code resources and showcase sites, you can discover even more code snippets to enhance your programming skills and take your coding to the next level.